Simple Logging Facade for Java (SLF4J) is an abstraction of different logging frameworks (eg. log4j, java.util.logging, commons logging etc.). This gives the developer an opportunity to plug-in desired logging framework at deployment time without changing the code.
To use SLF4J, it is required to include 3 jars SLF4J API (slf4j-api-x.x.x.jar), SLF4J bindings jar (eg. slf4j-log4j12-1.6.5.jar) and the actual logging framework (eg. log4j, java.util.logging etc).
SLF4J bindings is an adaptation layer between SLF4J API and the underlying logging framework that you wanted to use. Go through the following link to know which adaptation jar is required for which logging framework http://www.slf4j.org/manual.html#binding.
In this current example I have used java.util.logging as my underlying logging framework. I have put Adaptation layer / SLF4J bindings 'slf4j-jdk14-1.6.5.jar' required for java.util.logging.So in this case 'slf4j-jdk14-1.6.5.jar' will act as an adapter between SLF4J API and actual logging framework.Now, if you want to use log4j then add adapter 'slf4j-log4j12-1.6.5.jar' in classpath and configure log4j as per your requirement.You do not have to modify any code for the same.
Technologies used in this article :
1. Create a Java Project and a Class with 'main' method
Create a java project ('SLF4JHello') and a class ('SLF4JHello') in eclipse to run the 'Hello World!' sample code using SLF4j logging framework.Note:
To know how to create a java project in Eclipse IDE, go through the following tutorial "Java Hello World Example using Eclipse IDE".
Sample project structure is shown below
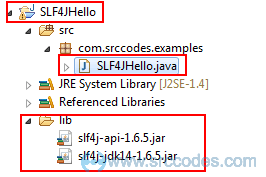
2. Copy slf4j jars
Create a 'lib' folder inside the project folder and copy the following jars 'slf4j-api-1.6.5.jar' and 'slf4j-jdk14-1.6.5.jar' to the newly created 'lib' folder. These jars are already included in the full source code provided with this tutorial in the 'Download Source Code' section.
3. Configure Build Path
Add slf4j jars to the build path of your project as shown below.
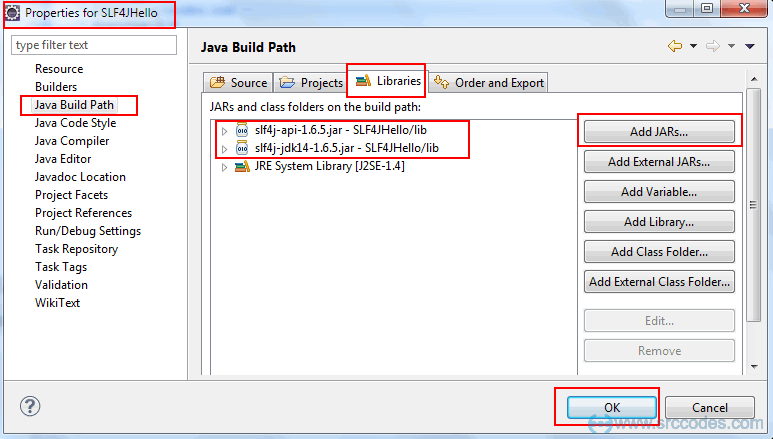
4. Write Code
Modify your code as per the following code.
File : SLF4JHello.java
package com.srccodes.examples;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* @author srccodes.com
* @version 1.0
*/
public class SLF4JHello {
private final Logger slf4jLogger = LoggerFactory.getLogger(SLF4JHello.class);
/**
* Print hello in log.
*
* @param name
*/
public void sayHello(String name) {
slf4jLogger.info("Hi, {}", name);
slf4jLogger.info("Welcome to the HelloWorld example of SLF4J");
}
/**
* @param args
*/
public static void main(String[] args) {
SLF4JHello slf4jHello = new SLF4JHello();
slf4jHello.sayHello("srccodes.com");
}
}
Note: Please refer the 'slf4j FAQ' link in the 'References' section to know the usage of '{}' and to know how it helps in performance optimization in logging.
5. Run Your Code
Right click on 'SLF4JHello.java' and select from context menu 'Run As' --> 'Java Application'.
6. Console Output
Your code will generate logs in the console.
Comments