I have been told that, medically, Cucumber can cause indigestion. Let's see...
What is Cucumber?
Cucumber is a testing framework that helps to bridge the gap between software developers and business managers. Tests are written in plain language based on the behavior-driven development (BDD) style of Given, When, Then which any layperson can understand. Cucumber allows software development teams describe how software should behave in plain text. The text is written in a business-readable domain-specific language and serves as documentation, automated tests and development-aid - all rolled into one format.
Why Cucumber?
Cucumber allows you to write feature documentation in Plain Text. It means you could sit with your Client or Business Analyst to write down the features to be built on your application. Hence proper use of this framework has a great potential to bridge the gap between business people and development people.
Components to be developed/set up
- Environment ( to be set up to run test cases)
- Feature File
- Step File
- Runner File with Glue Code
Let's briefly describe each of these:
1. Environment set up
Let's assume that the target application to be tested is a Java Application. All we need to do is to provide required jar files in appropriate path to integrate Cucumber with project. Here is the set of jar files that we need:
If the project is a Maven project then integration much easier. We just need to specify dependencies in Pom file as shown below:
For Maven project, conventionally, Feature Files are kept under src/test/resources and Step Files are kept under src/test/java. We can have any package hierarchy under these.
2. Feature File
Here we describe the behavior under test in Plain Text based on the behavior-driven development (BDD) style of Given, When, Then and And.
- Given
- provides context for the test scenario to be executed, such as the point in your application that the test occurs as well as any prerequisite data.
- When
- specifies the set of actions that triggers the test, such as user or subsystem actions.
- Then
- specifies the expected result of the test.
Feature file can also contains dummy data set (called Data Table) that will be used during test execution as input, expected output and all assertion purposes.
Here is an example Feature File
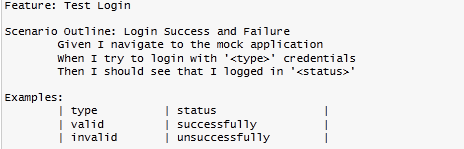
Note:
- Each of these Given When Then statements is called Step.
- A feature in non-trivial application can have multiple scenario outlines i.e. multiple flow. Hence we may need to specify multiple Scenario Outline elements in one feature file.
- We can provide "Placeholders" (e.g. '
') in an element and runtime values are supplied using Example element. Hence the Example elements (Data Table)supply the required runtime inputs to the test methods (or the step methods in Cucumber terminology) - Feature Files are written in Gherkin language. It strictly follows syntaxes. For example if we omit one "|" or ":" it throws InitializationError at runtime. "Feature", "Scenario Outline", "Example" are keywords. Playing with them leads to ParserException at runtime and tests will not run.
3. Step File
When Cucumber executes a Step in a Scenario it will look for a matching Step Definition to execute. These Step Definitions are written in Step File using languages like Java, Ruby and Python etc.
A Step Definition is a small piece of code with a (regex) pattern attached to it. The pattern is used to link the step definition to all the matching Steps and Cucumber will execute the code written inside Step Definition when it sees a Gherkin Step.
To understand how Step Definitions work, consider the following Step File which is written against previously shown Feature File.
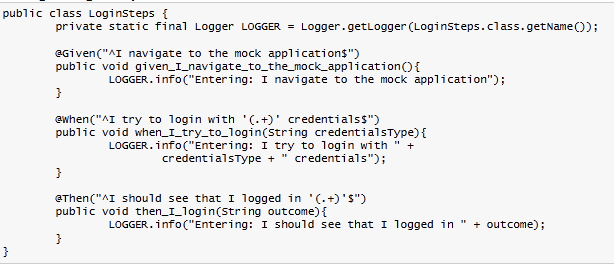
Above example may seem too trivial to understand the essence. So let me embed Feature File and Step File that we developed as a part of our project work.
Note:
- The method annotated with @When contains the core; here we need to mock the activity whose behavior we are going to test.
- The method annotated with @Then is the place where we are going to put our asserts against the outcomes of activities.
- Values supplied through Data Table of Feature File are assigned to the corresponding Step Definition method's arguments in execution time. Means to say 'valid' is assigned to 'credentialsType'.
- Verbose naming of step methods is only by convention, not mandatory. So a step method name can be "abc"; but the regex pattern must match for the method to be executed against a Step.
- The number of argument in the method signature also must match with the no of placeholders in Feature File's Step.
- If we specify two differently named methods with same regex pattern then, at runtime, error will occur (DuplicateStepException)
4. Runner File with Glue Code
We need a Runner File to run the test. Let's take an example to elaborate what it does:
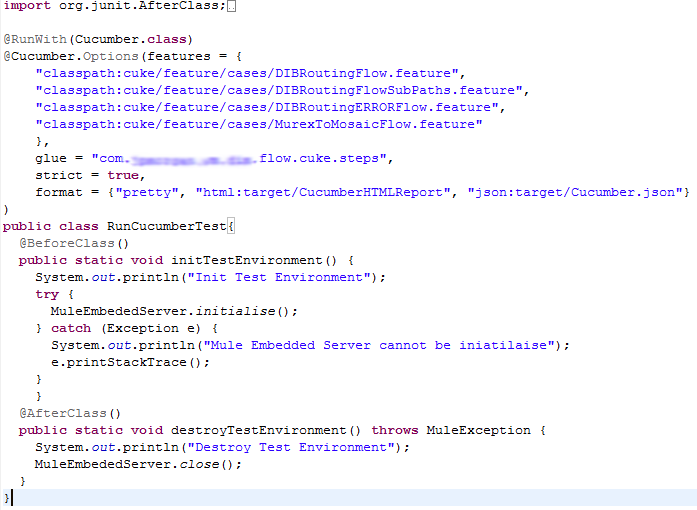
- It contains the location of Feature Files and the package containing the corresponding Step Files. Thus it glues the Feature Files with the Step Files and glues both with the cucumber runtime.
- It also specifies the format in which the test result will be rendered. For example here it is specified that output will be published both in HTML and JSON format.
- It also specifies the location and file name in which the test result will be published. For example here it is specified that output will be published in target folder and the names of the published files are CucumberHTMLReport and Cucumber.json.
- Here we can also provide methods for Initialization and Destruction of Test Environment just like we used to do for JUnit.
Note:
- As you can see from the glue code we need to specify the package for the Step Files (NOT the specific Java file name). It suggests that there is no One-To-One mapping between Feature File and Step File by their naming convention.
- Multiple feature file can be implemented by single Step File having methods with matching pattern for each of the steps in those Feature Files.
- Single Feature File can be implemented by multiple Step Files as the Step Methods can be distributed among those Step Files; only restriction is that all those Step Files are to be in the specified package.
- Matching Step Methods are searched across the specified package, Hence even if we specify two differently named methods with same regex pattern in two different Step Files in same package, then also at runtime error will occur (DuplicateStepException).
- Step Methods are reusable. Means to say, for same Steps written in different Step Scenarios or even different Feature Files (generally Given steps can be like that) we need to write only one Step Method.
How to Run?
- For running these test cases Standalone way, we can use any Eclipse IDE and right click on the Runner file and the Run As JUnit Test.
- For running these at build time and we are working with Maven project and we maintain the conventions as mentioned in Environment set up section then simple mvn install will execute all the Cucumber test cases at build time.
Comments