I have to observe lots of developers as part of my job description. I sit back and stare at what they do all the time, how they communicate to each other, how they express their ideas and how easily they get into a fight in a design discussion.
As per my experience, 80% of the design meetings do not live up to level of expectation because two developers are talking about the same implementation in two different approaches. Both of them has it's pros and cons and their designs can be combined into a far better framework only if their authors could understand each other.
Hence, the article below is one of numerous efforts to enable people understand each other when they are thinking to get a solution of any problem. At the same time we will try to suggest an approach to design.
Nevertheless, no two designs are same, but this article is not discussing the correct way :-)
The Plan
We will start with UMLs/DFDs and then we will ensure we put our thoughts enough clarified so that another developer does not need a discussion to get the essence of our plan.
Today, we will see how we can infer a very simple design from a problem statement. We will focus on the following:
- Action statement
- Scope of system
- Actors
- Entity
- Interaction
- Constraints
Problem Statement:
A customer asks for a pen to a shopkeeper. The shopkeeper asks for manufacturer preference or budget of the customer. The shopkeeper checks the price of the pen. Shop keeper also checks if there is any discount on this pen. Shopkeeper gives the pen to the customer. Customer pays the price. Shopkeeper pays back the change if any.
Solution:
The resulting level-0 DFD can be inferred as follows:
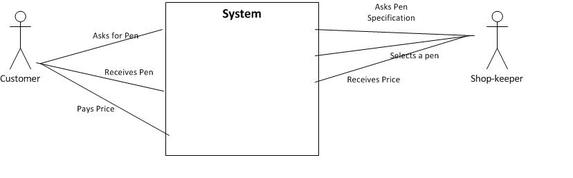
This can be further decomposed into Level 1 DFD as below:
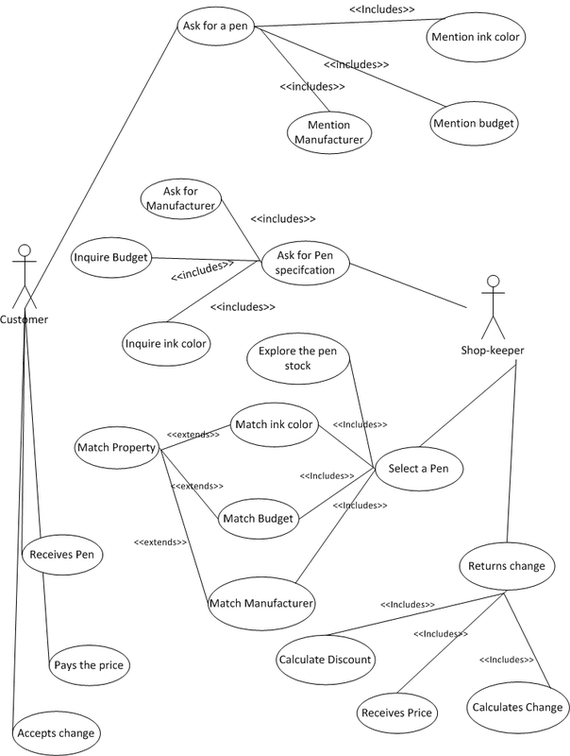
Now this diagram provides enough food for next level, which is identification of Entity.
Identification of Entity is crucial both for Database design or Object oriented code design.
Let's find the entities here:
- Customer
- Shop-keeper
- Pen (Off course)
- Stock of Pen
- Cash-box (Hidden but the essence of business)
Next step will be identifying the attributes and operations or in other words the variable and methods.
Class Diagram:
Let's design the Customer, Shop-keeper, Pen and Cash-box. Stock of Pen class will be derived afterwards as that depends on Pen.
Let's identify the Operations of Customer:
- Asks Pen
- Mention Ink color
- Mention budget
- Mention Manufacturer
- Pays Price
- Receives Pen
- Accepts Change
What are the attributes of the customer?
- Name (maybe)
- AADHAR No (in Indian context, futuristic thought)
- Budget
- No of Pen (He may want to have a collection)
Hence, the class diagram looks like as below:
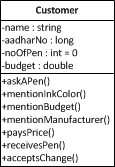
Subsequently the other classes will looks like:
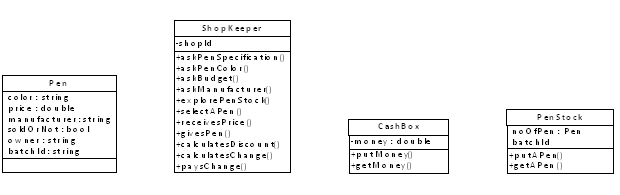
What next? ... Right you are , the sequence of operations.
Sequence Diagram:
Let's now design a flow of selecting a pen from pen stock by the shopkeeper.
Let's see do we have necessary information to start the design. Here we need required classes, operations, their sequences and finally some algorithm.
- Required Classes - We have the Actor, Shopkeeper class. We have operations like explorePenStock & selectAPen.
- We will first match Pen ink color, then budget and finally manufacturer.
- We may surely need some additional class or algorithm which we cannot think as of now but they will surface in next few minutes.
We need an operation which will match or compare budget, manufacturer or ink color. Now this is a generic behavior and may be needed for some other entities within this system. Hence, we will use an interface method to add that dimension in our class pen.
Interface MatchEntity{
public abstract matchSubject(Object a, Object b);
}
Note: This is something we do not often do while designing. As a designer you have to think about operations which can be of re-use. Now, the bad part is, nobody will tell you "this is the operation which can be re-used". Hence, use your intuition.
Now we have to deduce the algorithm to finalize our call sequence.
Draft Algorithm
- Shop-Keeper gathers Pen requirement, i.e. color, budget and manufacturer
- Customer provides the data
- Shop-keeper captures the data
- Load stock of pen
- Shop-keeper selects the pen
- Shop-keeper runs through his stock of pens
- Shop-keeper matches pen requirement with individual stock
- The first pen matching the requirement will be returned
- The details of the pen will be reported
Here is the Sequence Diagram as per our algorithm above:
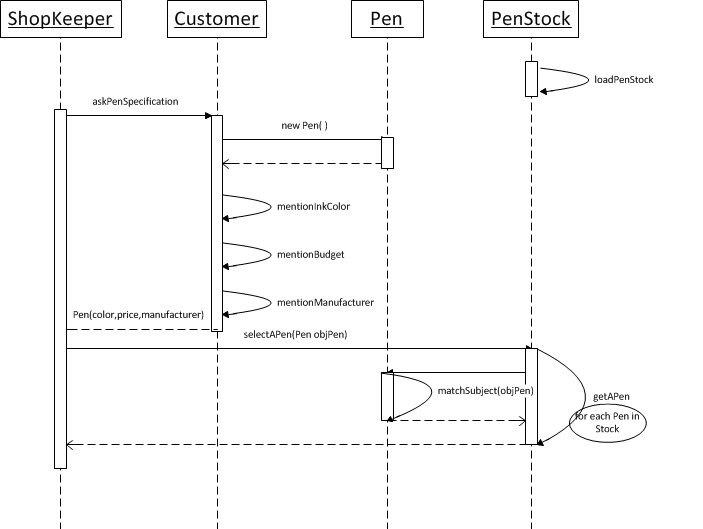
To ensure we understand the logic of matchSubject method we can write some pseudo-code:
Public Boolean matchSubject(Pen objPen){
If(objPen is not null){
If (objPen.price is equal to this.price){
If(objPen.manufacturer is equal to this.price){
If(objPen.inkColor is equal to this.inkColor){
Return true;
}
}
}
}
Return false;
}
We can also write the pseudo-code for selectAPen & getAPen method as below:
Public Pen selectAPen(Pen objPen){
Pen selectedPen = Penstock .getAPen(objPen);
Return selectedPen;
}
Public Pen getAPen(Pen objPen){
Pen returnedPen = null;
For each Pen pen in Penstock {
If(pen.matchSubject(objPen)){
returnedPen = pen;
Break;
}
}
Return returnedPen;
}
Hopefully I am able to present you an approach to represent your idea as a software design.
Kindly get in touch in case you have any suggestion or query.
Comments